|
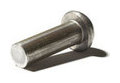 |
Rivet 4.1.0
|
Calculate missing \( E \), \( E_\perp \) etc. as complements to the total visible momentum.
More...
#include <MissingMomentum.hh>
|
| MissingMomentum (const FinalState &fs) |
| Canonical constructor taking a FinalState as argument.
|
|
| MissingMomentum (const Cut &c=Cuts::open()) |
| Default constructor with optional cut.
|
|
| RIVET_DEFAULT_PROJ_CLONE (MissingMomentum) |
| Clone on the heap.
|
|
void | clear () |
| Clear the projection results.
|
|
virtual Projection & | operator= (const Projection &)=delete |
| Import to avoid warnings about overload-hiding.
|
|
virtual void | reset () |
| Reset the projection. Smearing functions will be unchanged.
|
|
virtual std::string | name () const |
| Get the name of the projection.
|
|
bool | valid () const |
| Get the state of the projetion.
|
|
bool | failed () const |
| Get the state of the projetion.
|
|
void | markAsOwned () const |
| Mark this object as owned by a proj-handler.
|
|
|
const FourMomentum | visibleMomentum (double mass=0 *GeV) const |
| The vector-summed visible four-momentum in the event.
|
|
const FourMomentum | visibleMom (double mass=0 *GeV) const |
| Alias for visibleMomentum.
|
|
const FourMomentum | missingMomentum (double mass=0 *GeV) const |
| The missing four-momentum in the event, required to balance the final state.
|
|
const FourMomentum | missingMom (double mass=0 *GeV) const |
| Alias for missingMomentum.
|
|
|
- Note
- This may be what you want, even if the paper calls it "missing Et"!
|
const ThreeMomentum & | vectorPt () const |
| The vector-summed visible transverse momentum in the event, as a 3-vector with z=0.
|
|
const ThreeMomentum | vectorPtMiss () const |
| The vector missing transverse momentum in the event, as a 3-vector with z=0.
|
|
double | scalarPtMiss () const |
| The scalar value of missing transverse momentum in the event.
|
|
double | scalarPt () const |
| The scalar-summed visible transverse momentum in the event.
|
|
|
- Warning
- Despite the common names "MET" and "SET", what's often meant is the pT functions above!
|
const Vector3 & | vectorEt () const |
| The vector-summed visible transverse energy in the event, as a 3-vector with z=0.
|
|
const Vector3 | vectorEtMiss () const |
| The vector missing transverse energy in the event, as a 3-vector with z=0.
|
|
double | scalarEt () const |
| The scalar-summed visible transverse energy in the event.
|
|
|
- Note
- This may be what you want, even if the paper calls it "missing Et"!
|
const Vector3 | vectorMissingPt () const |
| Convenience vector MPT function.
|
|
const Vector3 | vectorMPT () const |
|
double | missingPt () const |
| The vector-summed missing transverse momentum in the event.
|
|
double | scalarSumPt () const |
| Alias for scalarPt.
|
|
|
- Warning
- Despite the common names "MET" and "SET", what's often meant is the pT functions above!
|
const Vector3 | vectorMissingEt () const |
| Convenience vector MET function.
|
|
const Vector3 | vectorMET () const |
|
double | missingEt () const |
| The vector-summed missing transverse energy in the event.
|
|
double | met () const |
| Alias for missingEt.
|
|
double | scalarSumEt () const |
| Alias for scalarEt.
|
|
double | set () const |
| Alias for scalarSumEt.
|
|
|
virtual unique_ptr< Projection > | clone () const =0 |
| Clone on the heap.
|
|
|
bool | before (const Projection &p) const |
|
|
std::set< ConstProjectionPtr > | getProjections () const |
| Get the contained projections, including recursion.
|
|
std::set< ConstProjectionPtr > | getImmediateChildProjections () const |
| Get the contained projections, excluding recursion.
|
|
bool | hasProjection (const std::string &name) const |
| Does this applier have a projection registered under the name name?
|
|
template<typename PROJ > |
const PROJ & | getProjection (const std::string &name) const |
|
const Projection & | getProjection (const std::string &name) const |
|
template<typename PROJ > |
const PROJ & | get (const std::string &name) const |
|
template<typename PROJ > |
const PROJ & | getProjectionFromDeclQueue (const std::string name) const |
|
|
template<typename PROJ = Projection> |
std::enable_if_t< std::is_base_of< Projection, PROJ >::value, const PROJ & > | apply (const Event &evt, const Projection &proj) const |
| Apply the supplied projection on event evt.
|
|
template<typename PROJ = Projection> |
std::enable_if_t< std::is_base_of< Projection, PROJ >::value, const PROJ & > | apply (const Event &evt, const PROJ &proj) const |
| Apply the supplied projection on event evt (user-facing alias).
|
|
template<typename PROJ = Projection> |
std::enable_if_t< std::is_base_of< Projection, PROJ >::value, const PROJ & > | apply (const Event &evt, const std::string &name) const |
| Apply the supplied projection on event evt (user-facing alias).
|
|
template<typename PROJ = Projection> |
std::enable_if_t< std::is_base_of< Projection, PROJ >::value, const PROJ & > | apply (const std::string &name, const Event &evt) const |
| Apply the supplied projection on event evt (convenience arg-reordering alias).
|
|
|
void | project (const Event &e) |
| Apply the projection to the event.
|
|
CmpState | compare (const Projection &p) const |
| Compare projections.
|
|
Log & | getLog () const |
| Get a Log object based on the getName() property of the calling projection object.
|
|
void | setName (const std::string &name) |
| Used by derived classes to set their name.
|
|
void | fail () |
| Set the projection in an unvalid state.
|
|
Cmp< Projection > | mkNamedPCmp (const Projection &otherparent, const std::string &pname) const |
|
Cmp< Projection > | mkPCmp (const Projection &otherparent, const std::string &pname) const |
|
ProjectionHandler & | getProjHandler () const |
| Get a reference to the ProjectionHandler for this thread.
|
|
void | setProjectionHandler (ProjectionHandler &projectionHandler) const |
|
|
template<typename PROJ > |
const PROJ & | declare (const PROJ &proj, const std::string &name) const |
| Register a contained projection (user-facing version)
|
|
template<typename PROJ > |
const PROJ & | declare (const std::string &name, const PROJ &proj) const |
| Register a contained projection (user-facing, arg-reordered version)
|
|
Calculate missing \( E \), \( E_\perp \) etc. as complements to the total visible momentum.
Project out the total visible energy vector, allowing missing \( E \), \( E_\perp \) etc. to be calculated. Final-state particle visibility restrictions are automatic, and the resulting visible/missing momentum vectors are over the whole event rather than over hard objects (jets + leptons) or specific to prompt invisibles.
◆ apply()
template<typename PROJ = Projection>
std::enable_if_t< std::is_base_of< Projection, PROJ >::value, const PROJ & > Rivet::ProjectionApplier::apply |
( |
const Event & |
evt, |
|
|
const Projection & |
proj |
|
) |
| const |
|
inlineinherited |
◆ before()
bool Rivet::Projection::before |
( |
const Projection & |
p | ) |
const |
|
inherited |
Determine whether this object should be ordered before the object p given as argument. If p is of a different class than this, the before() function of the corresponding type_info objects is used. Otherwise, if the objects are of the same class, the virtual compare(const Projection &) will be returned.
◆ clone()
virtual unique_ptr< Projection > Rivet::Projection::clone |
( |
| ) |
const |
|
pure virtualinherited |
◆ compare()
CmpState Rivet::MissingMomentum::compare |
( |
const Projection & |
p | ) |
const |
|
protectedvirtual |
◆ declare() [1/2]
template<typename PROJ >
const PROJ & Rivet::ProjectionApplier::declare |
( |
const PROJ & |
proj, |
|
|
const std::string & |
name |
|
) |
| const |
|
inlineprotectedinherited |
Register a contained projection (user-facing version)
- Todo:
- Add SFINAE to require that PROJ inherit from Projection
Referenced by Rivet::CentralEtHCM::CentralEtHCM(), Rivet::CentralityEstimator::CentralityEstimator(), Rivet::ChargedLeptons::ChargedLeptons(), Rivet::ALICE::CLMultiplicity< INNER >::CLMultiplicity(), Rivet::DISDiffHadron::DISDiffHadron(), Rivet::DISFinalState::DISFinalState(), Rivet::DISKinematics::DISKinematics(), Rivet::DISLepton::DISLepton(), Rivet::EventMixingBase::EventMixingBase(), Rivet::GammaGammaKinematics::GammaGammaKinematics(), Rivet::GammaGammaLeptons::GammaGammaLeptons(), Rivet::GammaGammaLeptons::GammaGammaLeptons(), Rivet::GeneratedCentrality::GeneratedCentrality(), Rivet::HadronicFinalState::HadronicFinalState(), Rivet::HeavyHadrons::HeavyHadrons(), Rivet::Hemispheres::Hemispheres(), Rivet::InvisibleFinalState::InvisibleFinalState(), Rivet::LeadingParticlesFinalState::LeadingParticlesFinalState(), Rivet::LossyFinalState< FILTER >::LossyFinalState(), Rivet::LossyFinalState< FILTER >::LossyFinalState(), Rivet::MC_pPbMinBiasTrigger::MC_pPbMinBiasTrigger(), Rivet::MC_SumETFwdPbCentrality::MC_SumETFwdPbCentrality(), Rivet::ATLAS::MinBiasTrigger::MinBiasTrigger(), MissingMomentum(), Rivet::NeutralFinalState::NeutralFinalState(), Rivet::NeutralFinalState::NeutralFinalState(), Rivet::NonHadronicFinalState::NonHadronicFinalState(), Rivet::ParisiTensor::ParisiTensor(), Rivet::PercentileProjection::PercentileProjection(), Rivet::PrimaryHadrons::PrimaryHadrons(), Rivet::PrimaryHadrons::PrimaryHadrons(), Rivet::SmearedMET::SmearedMET(), Rivet::SmearedMET::SmearedMET(), Rivet::SmearedMET::SmearedMET(), Rivet::Spherocity::Spherocity(), Rivet::ATLAS::SumET_PB_Centrality::SumET_PB_Centrality(), Rivet::ATLAS::SumET_PBPB_Centrality::SumET_PBPB_Centrality(), Rivet::TriggerCDFRun0Run1::TriggerCDFRun0Run1(), Rivet::TriggerCDFRun2::TriggerCDFRun2(), Rivet::UndressBeamLeptons::UndressBeamLeptons(), Rivet::ALICE::V0AndTrigger::V0AndTrigger(), Rivet::ALICE::V0Trigger< MODE >::V0Trigger(), Rivet::VetoedFinalState::VetoedFinalState(), Rivet::VisibleFinalState::VisibleFinalState(), Rivet::VisibleFinalState::VisibleFinalState(), Rivet::CentralityProjection::add(), Rivet::SimpleAnalysis::postInit(), Rivet::CentralityBinner< T, MDist >::setProjection(), and Rivet::VetoedFinalState::vetoFinalState().
◆ declare() [2/2]
template<typename PROJ >
const PROJ & Rivet::ProjectionApplier::declare |
( |
const std::string & |
name, |
|
|
const PROJ & |
proj |
|
) |
| const |
|
inlineprotectedinherited |
Register a contained projection (user-facing, arg-reordered version)
- Todo:
- Add SFINAE to require that PROJ inherit from Projection
◆ get()
template<typename PROJ >
const PROJ & Rivet::ProjectionApplier::get |
( |
const std::string & |
name | ) |
const |
|
inlineinherited |
Get the named projection, specifying return type via a template argument (user-facing alias). - Todo:
- Add SFINAE to require that PROJ inherit from Projection
◆ getProjection() [1/2]
template<typename PROJ >
const PROJ & Rivet::ProjectionApplier::getProjection |
( |
const std::string & |
name | ) |
const |
|
inlineinherited |
◆ getProjection() [2/2]
const Projection & Rivet::ProjectionApplier::getProjection |
( |
const std::string & |
name | ) |
const |
|
inlineinherited |
◆ getProjectionFromDeclQueue()
template<typename PROJ >
const PROJ & Rivet::ProjectionApplier::getProjectionFromDeclQueue |
( |
const std::string |
name | ) |
const |
|
inlineinherited |
Get a named projection from this projection appliers declqueue TODO for TP: Recursion?
References MSG_ERROR.
◆ missingMomentum()
const FourMomentum Rivet::MissingMomentum::missingMomentum |
( |
double |
mass = 0*GeV | ) |
const |
|
inline |
The missing four-momentum in the event, required to balance the final state.
- Note
- The optional mass argument is used to set a mass on the 4-vector. By default it is zero (since missing momentum is really a 3-momentum quantity: adding the E components of visible momenta just gives a huge mass)
- Todo:
- Change to return a 3-vector with no argument, a 4-vector if a mass arg given
References Rivet::mass(), Rivet::FourMomentum::reverse(), and visibleMomentum().
Referenced by missingMom().
◆ mkNamedPCmp()
Cmp< Projection > Rivet::Projection::mkNamedPCmp |
( |
const Projection & |
otherparent, |
|
|
const std::string & |
pname |
|
) |
| const |
|
protectedinherited |
Shortcut to make a named Cmp<Projection> comparison with the *this object automatically passed as one of the parent projections.
Referenced by Rivet::MC_SumETFwdPbCentrality::compare(), Rivet::MC_pPbMinBiasTrigger::compare(), Rivet::ATLAS::SumET_PB_Centrality::compare(), Rivet::ATLAS::SumET_PBPB_Centrality::compare(), Rivet::ATLAS::MinBiasTrigger::compare(), Rivet::BeamThrust::compare(), Rivet::CentralEtHCM::compare(), Rivet::DISFinalState::compare(), Rivet::EventMixingBase::compare(), Rivet::FParameter::compare(), Rivet::GammaGammaFinalState::compare(), Rivet::HeavyHadrons::compare(), Rivet::Hemispheres::compare(), Rivet::LossyFinalState< FILTER >::compare(), Rivet::PercentileProjection::compare(), Rivet::STAR_BES_Centrality::compare(), Rivet::BRAHMSCentrality::compare(), Rivet::Spherocity::compare(), Rivet::Thrust::compare(), Rivet::CentralityEstimator::compare(), and Rivet::GeneratedCentrality::compare().
◆ mkPCmp()
Cmp< Projection > Rivet::Projection::mkPCmp |
( |
const Projection & |
otherparent, |
|
|
const std::string & |
pname |
|
) |
| const |
|
protectedinherited |
◆ name()
virtual std::string Rivet::Projection::name |
( |
| ) |
const |
|
inlinevirtualinherited |
◆ operator=()
Import to avoid warnings about overload-hiding.
Reimplemented from Rivet::METFinder.
◆ project()
void Rivet::MissingMomentum::project |
( |
const Event & |
e | ) |
|
|
protectedvirtual |
◆ reset()
virtual void Rivet::METFinder::reset |
( |
| ) |
|
|
inlinevirtualinherited |
Reset the projection. Smearing functions will be unchanged.
Reimplemented in Rivet::SmearedMET.
◆ scalarEt()
double Rivet::MissingMomentum::scalarEt |
( |
| ) |
const |
|
inlinevirtual |
The scalar-summed visible transverse energy in the event.
Implements Rivet::METFinder.
◆ scalarPt()
double Rivet::MissingMomentum::scalarPt |
( |
| ) |
const |
|
inlinevirtual |
The scalar-summed visible transverse momentum in the event.
Implements Rivet::METFinder.
◆ setProjectionHandler()
void Rivet::ProjectionApplier::setProjectionHandler |
( |
ProjectionHandler & |
projectionHandler | ) |
const |
|
protectedinherited |
- Todo:
- AB: Add Doxygen comment, follow surrounding coding style
◆ vectorEt()
const Vector3 & Rivet::MissingMomentum::vectorEt |
( |
| ) |
const |
|
inlinevirtual |
The vector-summed visible transverse energy in the event, as a 3-vector with z=0.
- Note
- Reverse this vector with operator- to get the missing ET vector.
Implements Rivet::METFinder.
Referenced by vectorEtMiss().
◆ vectorPt()
The vector-summed visible transverse momentum in the event, as a 3-vector with z=0.
- Note
- Reverse this vector with operator- to get the missing pT vector.
Implements Rivet::METFinder.
Referenced by vectorPtMiss().
◆ visibleMomentum()
const FourMomentum Rivet::MissingMomentum::visibleMomentum |
( |
double |
mass = 0 *GeV | ) |
const |
The vector-summed visible four-momentum in the event.
- Note
- Reverse this vector with .reverse() to get the missing momentum vector.
-
The optional mass argument is used to set a mass on the 4-vector. By default it is zero (since missing momentum is really a 3-momentum quantity: adding the E components of visible momenta just gives a huge mass)
- Todo:
- Change to return a 3-vector with no argument, a 4-vector if a mass arg given
Referenced by missingMomentum(), and visibleMom().
The documentation for this class was generated from the following file:
|