|
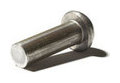 |
Rivet 4.1.0
|
4#include "Rivet/Tools/Cuts.fhh"
17 template < typename ClassToCheck>
18 bool accept( const ClassToCheck&) const;
22 template < typename ClassToCheck>
23 bool operator () ( const ClassToCheck& x) const { return accept(x); }
26 virtual bool operator == ( const Cut&) const = 0;
29 virtual std::string toString() const = 0;
38 virtual bool _accept( const CuttableBase&) const = 0;
44 inline bool operator == ( const Cut& a, const Cut& b) { return *a == b; }
58 enum Quantity { pT=0, pt=0, Et=1, et=1, E=2, energy=2,
59 mass, rap, absrap, eta, abseta, phi,
60 pid, abspid, charge, abscharge, charge3, abscharge3, pz };
65 extern const Cut& OPEN;
66 extern const Cut& NOCUT;
70 Cut range( Quantity, double m, double n);
71 inline Cut ptIn( double m, double n) { return range(pT, m,n); }
72 inline Cut etIn( double m, double n) { return range(Et, m,n); }
73 inline Cut energyIn( double m, double n) { return range(energy, m,n); }
74 inline Cut massIn( double m, double n) { return range(mass, m,n); }
75 inline Cut rapIn( double m, double n) { return range(rap, m,n); }
76 inline Cut absrapIn( double m, double n) { return range(absrap, m,n); }
77 inline Cut etaIn( double m, double n) { return range(eta, m,n); }
78 inline Cut absetaIn( double m, double n) { return range(abseta, m,n); }
96 inline Cut operator != ( Cuts::Quantity qty, int i) { return qty != double(i); }
99 inline Cut operator < ( Cuts::Quantity qty, int i) { return qty < double(i); }
100 inline Cut operator > ( Cuts::Quantity qty, int i) { return qty > double(i); }
101 inline Cut operator <= ( Cuts::Quantity qty, int i) { return qty <= double(i); }
102 inline Cut operator >= ( Cuts::Quantity qty, int i) { return qty >= double(i); }
133 inline std::ostream& operator << (std::ostream& os, const Cut& cptr) {
134 os << cptr->toString();
const Cut & open() Fully open cut singleton, accepts everything.
Quantity Available categories of cut objects. Definition Cuts.hh:58
Definition MC_CENT_PPB_Projections.hh:10
Cut operator|(const Cut &aptr, const Cut &bptr) Logical OR operation on two cuts.
Cut operator!(const Cut &cptr) Logical NOT operation on a cut.
bool operator==(const Cut &a, const Cut &b) Compare two cuts for equality, forwards to the cut-specific implementation. Definition Cuts.hh:44
Cut operator&&(const Cut &aptr, const Cut &bptr)
Cut operator^(const Cut &aptr, const Cut &bptr) Logical XOR operation on two cuts.
std::ostream & operator<<(std::ostream &os, const AnalysisInfo &ai) Stream an AnalysisInfo as a text description. Definition AnalysisInfo.hh:362
Cut operator&(const Cut &aptr, const Cut &bptr) Logical AND operation on two cuts.
Cut operator,(const Cut &, const Cut &)=delete
Cut operator~(const Cut &cptr) Logical NOT operation on a cut.
std::string toString(const AnalysisInfo &ai) String representation.
Cut operator||(const Cut &aptr, const Cut &bptr)
|