|
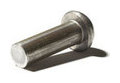 |
Rivet 3.1.6
|
|
template<typename V4TYPE , typename std::enable_if< HasXYZT< V4TYPE >::value, int >::type DUMMY = 0> |
| Rivet::FourVector::FourVector (const V4TYPE &other) |
|
| Rivet::FourVector::FourVector (const Vector< 4 > &other) |
|
| Rivet::FourVector::FourVector (const double t, const double x, const double y, const double z) |
|
double | Rivet::FourVector::t () const |
|
double | Rivet::FourVector::t2 () const |
|
FourVector & | Rivet::FourVector::setT (const double t) |
|
double | Rivet::FourVector::x () const |
|
double | Rivet::FourVector::x2 () const |
|
FourVector & | Rivet::FourVector::setX (const double x) |
|
double | Rivet::FourVector::y () const |
|
double | Rivet::FourVector::y2 () const |
|
FourVector & | Rivet::FourVector::setY (const double y) |
|
double | Rivet::FourVector::z () const |
|
double | Rivet::FourVector::z2 () const |
|
FourVector & | Rivet::FourVector::setZ (const double z) |
|
double | Rivet::FourVector::invariant () const |
|
bool | Rivet::FourVector::isNull () const |
|
double | Rivet::FourVector::angle (const FourVector &v) const |
| Angle between this vector and another.
|
|
double | Rivet::FourVector::angle (const Vector3 &v3) const |
| Angle between this vector and another (3-vector)
|
|
double | Rivet::FourVector::polarRadius2 () const |
| Mod-square of the projection of the 3-vector on to the \( x-y \) plane This is a more efficient function than polarRadius , as it avoids the square root. Use it if you only need the squared value, or e.g. an ordering by magnitude.
|
|
double | Rivet::FourVector::perp2 () const |
| Synonym for polarRadius2.
|
|
double | Rivet::FourVector::rho2 () const |
| Synonym for polarRadius2.
|
|
double | Rivet::FourVector::polarRadius () const |
| Magnitude of projection of 3-vector on to the \( x-y \) plane.
|
|
double | Rivet::FourVector::perp () const |
| Synonym for polarRadius.
|
|
double | Rivet::FourVector::rho () const |
| Synonym for polarRadius.
|
|
Vector3 | Rivet::FourVector::polarVec () const |
| Projection of 3-vector on to the \( x-y \) plane.
|
|
Vector3 | Rivet::FourVector::perpVec () const |
| Synonym for polarVec.
|
|
Vector3 | Rivet::FourVector::rhoVec () const |
| Synonym for polarVec.
|
|
double | Rivet::FourVector::azimuthalAngle (const PhiMapping mapping=ZERO_2PI) const |
| Angle subtended by the 3-vector's projection in x-y and the x-axis.
|
|
double | Rivet::FourVector::phi (const PhiMapping mapping=ZERO_2PI) const |
| Synonym for azimuthalAngle.
|
|
double | Rivet::FourVector::polarAngle () const |
| Angle subtended by the 3-vector and the z-axis.
|
|
double | Rivet::FourVector::theta () const |
| Synonym for polarAngle.
|
|
double | Rivet::FourVector::pseudorapidity () const |
| Pseudorapidity (defined purely by the 3-vector components)
|
|
double | Rivet::FourVector::eta () const |
| Synonym for pseudorapidity.
|
|
double | Rivet::FourVector::abspseudorapidity () const |
| Get the \( |\eta| \) directly.
|
|
double | Rivet::FourVector::abseta () const |
| Get the \( |\eta| \) directly (alias).
|
|
Vector3 | Rivet::FourVector::vector3 () const |
| Get the spatial part of the 4-vector as a 3-vector.
|
|
| Rivet::FourVector::operator Vector3 () const |
| Implicit cast to a 3-vector.
|
|
double | Rivet::FourVector::contract (const FourVector &v) const |
| Contract two 4-vectors, with metric signature (+ - - -).
|
|
double | Rivet::FourVector::dot (const FourVector &v) const |
| Contract two 4-vectors, with metric signature (+ - - -).
|
|
double | Rivet::FourVector::operator* (const FourVector &v) const |
| Contract two 4-vectors, with metric signature (+ - - -).
|
|
FourVector & | Rivet::FourVector::operator*= (double a) |
| Multiply by a scalar.
|
|
FourVector & | Rivet::FourVector::operator/= (double a) |
| Divide by a scalar.
|
|
FourVector & | Rivet::FourVector::operator+= (const FourVector &v) |
| Add to this 4-vector.
|
|
FourVector & | Rivet::FourVector::operator-= (const FourVector &v) |
| Subtract from this 4-vector. NB time as well as space components are subtracted.
|
|
FourVector | Rivet::FourVector::operator- () const |
| Multiply all components (space and time) by -1.
|
|
FourVector | Rivet::FourVector::reverse () const |
| Multiply space components only by -1.
|
|
template<typename V4TYPE , typename std::enable_if< HasXYZT< V4TYPE >::value, int >::type DUMMY = 0> |
| Rivet::FourMomentum::FourMomentum (const V4TYPE &other) |
|
| Rivet::FourMomentum::FourMomentum (const Vector< 4 > &other) |
|
| Rivet::FourMomentum::FourMomentum (const double E, const double px, const double py, const double pz) |
|
|
FourMomentum & | Rivet::FourMomentum::setE (double E) |
| Set energy \( E \) (time component of momentum).
|
|
FourMomentum & | Rivet::FourMomentum::setPx (double px) |
| Set x-component of momentum \( p_x \).
|
|
FourMomentum & | Rivet::FourMomentum::setPy (double py) |
| Set y-component of momentum \( p_y \).
|
|
FourMomentum & | Rivet::FourMomentum::setPz (double pz) |
| Set z-component of momentum \( p_z \).
|
|
FourMomentum & | Rivet::FourMomentum::setPE (double px, double py, double pz, double E) |
| Set the p coordinates and energy simultaneously.
|
|
FourMomentum & | Rivet::FourMomentum::setXYZE (double px, double py, double pz, double E) |
| Alias for setPE.
|
|
FourMomentum & | Rivet::FourMomentum::setPM (double px, double py, double pz, double mass) |
| Set the p coordinates and mass simultaneously.
|
|
FourMomentum & | Rivet::FourMomentum::setXYZM (double px, double py, double pz, double mass) |
| Alias for setPM.
|
|
FourMomentum & | Rivet::FourMomentum::setEtaPhiME (double eta, double phi, double mass, double E) |
|
FourMomentum & | Rivet::FourMomentum::setEtaPhiMPt (double eta, double phi, double mass, double pt) |
|
FourMomentum & | Rivet::FourMomentum::setRapPhiME (double y, double phi, double mass, double E) |
|
FourMomentum & | Rivet::FourMomentum::setRapPhiMPt (double y, double phi, double mass, double pt) |
|
FourMomentum & | Rivet::FourMomentum::setThetaPhiME (double theta, double phi, double mass, double E) |
|
FourMomentum & | Rivet::FourMomentum::setThetaPhiMPt (double theta, double phi, double mass, double pt) |
|
FourMomentum & | Rivet::FourMomentum::setPtPhiME (double pt, double phi, double mass, double E) |
|
|
double | Rivet::FourMomentum::E () const |
| Get energy \( E \) (time component of momentum).
|
|
double | Rivet::FourMomentum::E2 () const |
| Get energy-squared \( E^2 \).
|
|
double | Rivet::FourMomentum::px () const |
| Get x-component of momentum \( p_x \).
|
|
double | Rivet::FourMomentum::px2 () const |
| Get x-squared \( p_x^2 \).
|
|
double | Rivet::FourMomentum::py () const |
| Get y-component of momentum \( p_y \).
|
|
double | Rivet::FourMomentum::py2 () const |
| Get y-squared \( p_y^2 \).
|
|
double | Rivet::FourMomentum::pz () const |
| Get z-component of momentum \( p_z \).
|
|
double | Rivet::FourMomentum::pz2 () const |
| Get z-squared \( p_z^2 \).
|
|
double | Rivet::FourMomentum::mass () const |
| Get the mass \( m = \sqrt{E^2 - p^2} \) (the Lorentz self-invariant). More...
|
|
double | Rivet::FourMomentum::mass2 () const |
| Get the squared mass \( m^2 = E^2 - p^2 \) (the Lorentz self-invariant).
|
|
Vector3 | Rivet::FourMomentum::p3 () const |
| Get 3-momentum part, \( p \).
|
|
double | Rivet::FourMomentum::p () const |
| Get the modulus of the 3-momentum.
|
|
double | Rivet::FourMomentum::p2 () const |
| Get the modulus-squared of the 3-momentum.
|
|
double | Rivet::FourMomentum::rapidity () const |
| Calculate the rapidity.
|
|
double | Rivet::FourMomentum::rap () const |
| Alias for rapidity.
|
|
double | Rivet::FourMomentum::absrapidity () const |
| Absolute rapidity.
|
|
double | Rivet::FourMomentum::absrap () const |
| Absolute rapidity.
|
|
Vector3 | Rivet::FourMomentum::pTvec () const |
| Calculate the transverse momentum vector \( \vec{p}_T \).
|
|
Vector3 | Rivet::FourMomentum::ptvec () const |
| Synonym for pTvec.
|
|
double | Rivet::FourMomentum::pT2 () const |
| Calculate the squared transverse momentum \( p_T^2 \).
|
|
double | Rivet::FourMomentum::pt2 () const |
| Calculate the squared transverse momentum \( p_T^2 \).
|
|
double | Rivet::FourMomentum::pT () const |
| Calculate the transverse momentum \( p_T \).
|
|
double | Rivet::FourMomentum::pt () const |
| Calculate the transverse momentum \( p_T \).
|
|
double | Rivet::FourMomentum::Et2 () const |
| Calculate the transverse energy \( E_T^2 = E^2 \sin^2{\theta} \).
|
|
double | Rivet::FourMomentum::Et () const |
| Calculate the transverse energy \( E_T = E \sin{\theta} \).
|
|
|
FourMomentum & | Rivet::FourMomentum::operator*= (double a) |
| Multiply by a scalar.
|
|
FourMomentum & | Rivet::FourMomentum::operator/= (double a) |
| Divide by a scalar.
|
|
FourMomentum & | Rivet::FourMomentum::operator+= (const FourMomentum &v) |
| Add to this 4-vector. NB time as well as space components are added.
|
|
FourMomentum & | Rivet::FourMomentum::operator-= (const FourMomentum &v) |
| Subtract from this 4-vector. NB time as well as space components are subtracted.
|
|
FourMomentum | Rivet::FourMomentum::operator- () const |
| Multiply all components (time and space) by -1.
|
|
FourMomentum | Rivet::FourMomentum::reverse () const |
| Multiply space components only by -1.
|
|
|
static FourMomentum | Rivet::FourMomentum::mkXYZE (double px, double py, double pz, double E) |
| Make a vector from (px,py,pz,E) coordinates.
|
|
static FourMomentum | Rivet::FourMomentum::mkXYZM (double px, double py, double pz, double mass) |
| Make a vector from (px,py,pz) coordinates and the mass.
|
|
static FourMomentum | Rivet::FourMomentum::mkEtaPhiME (double eta, double phi, double mass, double E) |
| Make a vector from (eta,phi,energy) coordinates and the mass.
|
|
static FourMomentum | Rivet::FourMomentum::mkEtaPhiMPt (double eta, double phi, double mass, double pt) |
| Make a vector from (eta,phi,pT) coordinates and the mass.
|
|
static FourMomentum | Rivet::FourMomentum::mkRapPhiME (double y, double phi, double mass, double E) |
| Make a vector from (y,phi,energy) coordinates and the mass.
|
|
static FourMomentum | Rivet::FourMomentum::mkRapPhiMPt (double y, double phi, double mass, double pt) |
| Make a vector from (y,phi,pT) coordinates and the mass.
|
|
static FourMomentum | Rivet::FourMomentum::mkThetaPhiME (double theta, double phi, double mass, double E) |
| Make a vector from (theta,phi,energy) coordinates and the mass.
|
|
static FourMomentum | Rivet::FourMomentum::mkThetaPhiMPt (double theta, double phi, double mass, double pt) |
| Make a vector from (theta,phi,pT) coordinates and the mass.
|
|
static FourMomentum | Rivet::FourMomentum::mkPtPhiME (double pt, double phi, double mass, double E) |
| Make a vector from (pT,phi,energy) coordinates and the mass.
|
|
◆ beta()
double Rivet::FourMomentum::beta |
( |
| ) |
const |
|
inline |
◆ betaVec()
Vector3 Rivet::FourMomentum::betaVec |
( |
| ) |
const |
|
inline |
◆ gamma()
double Rivet::FourMomentum::gamma |
( |
| ) |
const |
|
inline |
◆ gammaVec()
Vector3 Rivet::FourMomentum::gammaVec |
( |
| ) |
const |
|
inline |
◆ mass()
double Rivet::FourMomentum::mass |
( |
| ) |
const |
|
inline |
Get the mass \( m = \sqrt{E^2 - p^2} \) (the Lorentz self-invariant).
For spacelike momenta, the mass will be -sqrt(|mass2|).
References Rivet::FourMomentum::mass2(), and Rivet::sign().
Referenced by Rivet::cmpMomByAscMass(), Rivet::cmpMomByMass(), Rivet::ParticleBase::mass(), Rivet::FourMomentum::mkEtaPhiME(), Rivet::FourMomentum::mkEtaPhiMPt(), Rivet::FourMomentum::mkPtPhiME(), Rivet::FourMomentum::mkRapPhiME(), Rivet::FourMomentum::mkRapPhiMPt(), Rivet::FourMomentum::mkThetaPhiME(), Rivet::FourMomentum::mkThetaPhiMPt(), Rivet::FourMomentum::mkXYZM(), Rivet::FourMomentum::setEtaPhiME(), Rivet::FourMomentum::setEtaPhiMPt(), Rivet::FourMomentum::setPM(), Rivet::FourMomentum::setPtPhiME(), Rivet::FourMomentum::setRapPhiME(), Rivet::FourMomentum::setRapPhiMPt(), Rivet::FourMomentum::setThetaPhiME(), Rivet::FourMomentum::setThetaPhiMPt(), and Rivet::FourMomentum::setXYZM().
◆ setEtaPhiME()
FourMomentum & Rivet::FourMomentum::setEtaPhiME |
( |
double |
eta, |
|
|
double |
phi, |
|
|
double |
mass, |
|
|
double |
E |
|
) |
| |
|
inline |
◆ setEtaPhiMPt()
FourMomentum & Rivet::FourMomentum::setEtaPhiMPt |
( |
double |
eta, |
|
|
double |
phi, |
|
|
double |
mass, |
|
|
double |
pt |
|
) |
| |
|
inline |
Set the vector state from (eta,phi,pT) coordinates and the mass
eta = -ln(tan(theta/2)) -> theta = 2 atan(exp(-eta))
References Rivet::FourMomentum::E(), Rivet::FourVector::eta(), Rivet::FourMomentum::mass(), Rivet::FourMomentum::p(), Rivet::FourVector::phi(), Rivet::FourMomentum::pt(), Rivet::FourMomentum::setThetaPhiME(), Rivet::sqr(), and Rivet::FourVector::theta().
Referenced by Rivet::FourMomentum::mkEtaPhiMPt().
◆ setPtPhiME()
FourMomentum & Rivet::FourMomentum::setPtPhiME |
( |
double |
pt, |
|
|
double |
phi, |
|
|
double |
mass, |
|
|
double |
E |
|
) |
| |
|
inline |
Set the vector state from (pT,phi,energy) coordinates and the mass
pz = sqrt(E^2 - mass^2 - pt^2)
References Rivet::FourMomentum::E(), Rivet::FourMomentum::mass(), Rivet::FourVector::phi(), Rivet::FourMomentum::pt(), Rivet::FourMomentum::px(), Rivet::FourMomentum::py(), Rivet::FourMomentum::pz(), Rivet::FourMomentum::setPE(), and Rivet::sqr().
Referenced by Rivet::FourMomentum::mkPtPhiME().
◆ setRapPhiME()
FourMomentum & Rivet::FourMomentum::setRapPhiME |
( |
double |
y, |
|
|
double |
phi, |
|
|
double |
mass, |
|
|
double |
E |
|
) |
| |
|
inline |
Set the vector state from (y,phi,energy) coordinates and the mass
y = 0.5 * ln((E+pz)/(E-pz)) -> (E^2 - pz^2) exp(2y) = (E+pz)^2 & (E^2 - pz^2) exp(-2y) = (E-pz)^2 -> E = sqrt(pt^2 + m^2) cosh(y) -> pz = sqrt(pt^2 + m^2) sinh(y) -> sqrt(pt^2 + m^2) = E / cosh(y)
References Rivet::FourMomentum::E(), Rivet::FourMomentum::mass(), Rivet::FourVector::phi(), Rivet::FourMomentum::pt(), Rivet::FourMomentum::px(), Rivet::FourMomentum::py(), Rivet::FourMomentum::pz(), Rivet::FourMomentum::setPE(), and Rivet::sqr().
Referenced by Rivet::FourMomentum::mkRapPhiME(), and Rivet::FourMomentum::setRapPhiMPt().
◆ setRapPhiMPt()
FourMomentum & Rivet::FourMomentum::setRapPhiMPt |
( |
double |
y, |
|
|
double |
phi, |
|
|
double |
mass, |
|
|
double |
pt |
|
) |
| |
|
inline |
◆ setThetaPhiME()
FourMomentum & Rivet::FourMomentum::setThetaPhiME |
( |
double |
theta, |
|
|
double |
phi, |
|
|
double |
mass, |
|
|
double |
E |
|
) |
| |
|
inline |
Set the vector state from (theta,phi,energy) coordinates and the mass
p = sqrt(E^2 - mass^2) pz = p cos(theta) pt = p sin(theta)
References Rivet::FourMomentum::E(), Rivet::FourMomentum::mass(), Rivet::FourMomentum::p(), Rivet::FourVector::phi(), Rivet::FourMomentum::pt(), Rivet::FourMomentum::px(), Rivet::FourMomentum::py(), Rivet::FourMomentum::pz(), Rivet::FourMomentum::setPE(), Rivet::sqr(), and Rivet::FourVector::theta().
Referenced by Rivet::FourMomentum::mkThetaPhiME(), Rivet::FourMomentum::setEtaPhiME(), and Rivet::FourMomentum::setEtaPhiMPt().
◆ setThetaPhiMPt()
FourMomentum & Rivet::FourMomentum::setThetaPhiMPt |
( |
double |
theta, |
|
|
double |
phi, |
|
|
double |
mass, |
|
|
double |
pt |
|
) |
| |
|
inline |
Set the vector state from (theta,phi,pT) coordinates and the mass
p = pt / sin(theta) pz = p cos(theta) E = sqrt(p^2 + mass^2)
References Rivet::FourMomentum::E(), Rivet::FourMomentum::mass(), Rivet::FourMomentum::p(), Rivet::FourVector::phi(), Rivet::FourMomentum::pt(), Rivet::FourMomentum::px(), Rivet::FourMomentum::py(), Rivet::FourMomentum::pz(), Rivet::FourMomentum::setPE(), Rivet::sqr(), and Rivet::FourVector::theta().
Referenced by Rivet::FourMomentum::mkThetaPhiMPt().
|