|
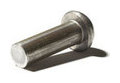 |
Rivet 3.1.6
|
|
void | Rivet::Analysis::scale (CounterPtr cnt, CounterAdapter factor) |
| Multiplicatively scale the given counter, cnt, by factor factor.
|
|
void | Rivet::Analysis::scale (const std::vector< CounterPtr > &cnts, CounterAdapter factor) |
|
template<typename T > |
void | Rivet::Analysis::scale (const std::map< T, CounterPtr > &maps, CounterAdapter factor) |
| Iteratively scale the counters in the map maps, by factor factor.
|
|
template<std::size_t array_size> |
void | Rivet::Analysis::scale (const CounterPtr(&cnts)[array_size], CounterAdapter factor) |
|
void | Rivet::Analysis::normalize (Histo1DPtr histo, CounterAdapter norm=1.0, bool includeoverflows=true) |
| Normalize the given histogram, histo, to area = norm.
|
|
void | Rivet::Analysis::normalize (const std::vector< Histo1DPtr > &histos, CounterAdapter norm=1.0, bool includeoverflows=true) |
|
template<typename T > |
void | Rivet::Analysis::normalize (const std::map< T, Histo1DPtr > &maps, CounterAdapter norm=1.0, bool includeoverflows=true) |
| Normalize the histograms in map, maps, to area = norm.
|
|
template<std::size_t array_size> |
void | Rivet::Analysis::normalize (const Histo1DPtr(&histos)[array_size], CounterAdapter norm=1.0, bool includeoverflows=true) |
|
void | Rivet::Analysis::barchart (Histo1DPtr h, Scatter2DPtr s, bool usefocus=false) const |
|
void | Rivet::Analysis::divide (CounterPtr c1, CounterPtr c2, Scatter1DPtr s) const |
|
void | Rivet::Analysis::divide (const YODA::Counter &c1, const YODA::Counter &c2, Scatter1DPtr s) const |
|
void | Rivet::Analysis::divide (const YODA::Histo1D &h1, const YODA::Histo1D &h2, Scatter2DPtr s) const |
|
void | Rivet::Analysis::divide (const YODA::Profile1D &p1, const YODA::Profile1D &p2, Scatter2DPtr s) const |
|
void | Rivet::Analysis::divide (const YODA::Histo2D &h1, const YODA::Histo2D &h2, Scatter3DPtr s) const |
|
void | Rivet::Analysis::divide (const YODA::Profile2D &p1, const YODA::Profile2D &p2, Scatter3DPtr s) const |
|
void | Rivet::Analysis::efficiency (Histo1DPtr h1, Histo1DPtr h2, Scatter2DPtr s) const |
|
void | Rivet::Analysis::efficiency (const YODA::Histo1D &h1, const YODA::Histo1D &h2, Scatter2DPtr s) const |
|
void | Rivet::Analysis::asymm (Histo1DPtr h1, Histo1DPtr h2, Scatter2DPtr s) const |
|
void | Rivet::Analysis::asymm (const YODA::Histo1D &h1, const YODA::Histo1D &h2, Scatter2DPtr s) const |
|
void | Rivet::Analysis::integrate (Histo1DPtr h, Scatter2DPtr s) const |
|
void | Rivet::Analysis::integrate (const Histo1D &h, Scatter2DPtr s) const |
|
- Todo:
- Should really be protected: only public to keep BinnedHistogram happy for now...
◆ asymm() [1/2]
void Rivet::Analysis::asymm |
( |
const YODA::Histo1D & |
h1, |
|
|
const YODA::Histo1D & |
h2, |
|
|
Scatter2DPtr |
s |
|
) |
| const |
Helper for histogram asymmetry calculation.
- Note
- Assigns to the (already registered) output scatter, s. Preserves the path information of the target.
◆ asymm() [2/2]
void Rivet::Analysis::asymm |
( |
Histo1DPtr |
h1, |
|
|
Histo1DPtr |
h2, |
|
|
Scatter2DPtr |
s |
|
) |
| const |
Helper for histogram asymmetry calculation.
- Note
- Assigns to the (already registered) output scatter, s. Preserves the path information of the target.
◆ barchart()
void Rivet::Analysis::barchart |
( |
Histo1DPtr |
h, |
|
|
Scatter2DPtr |
s, |
|
|
bool |
usefocus = false |
|
) |
| const |
- Todo:
- Add in-place conversions
Helper for histogram conversion to an inert scatter type
- Note
- Assigns to the (already registered) output scatter, s. Preserves the path information of the target.
◆ divide() [1/6]
void Rivet::Analysis::divide |
( |
const YODA::Counter & |
c1, |
|
|
const YODA::Counter & |
c2, |
|
|
Scatter1DPtr |
s |
|
) |
| const |
Helper for histogram division with raw YODA objects.
- Note
- Assigns to the (already registered) output scatter, s. Preserves the path information of the target.
◆ divide() [2/6]
void Rivet::Analysis::divide |
( |
const YODA::Histo1D & |
h1, |
|
|
const YODA::Histo1D & |
h2, |
|
|
Scatter2DPtr |
s |
|
) |
| const |
Helper for histogram division with raw YODA objects.
- Note
- Assigns to the (already registered) output scatter, s. Preserves the path information of the target.
◆ divide() [3/6]
void Rivet::Analysis::divide |
( |
const YODA::Histo2D & |
h1, |
|
|
const YODA::Histo2D & |
h2, |
|
|
Scatter3DPtr |
s |
|
) |
| const |
Helper for 2D histogram division with raw YODA objects.
- Note
- Assigns to the (already registered) output scatter, s. Preserves the path information of the target.
◆ divide() [4/6]
void Rivet::Analysis::divide |
( |
const YODA::Profile1D & |
p1, |
|
|
const YODA::Profile1D & |
p2, |
|
|
Scatter2DPtr |
s |
|
) |
| const |
Helper for profile histogram division with raw YODA objects.
- Note
- Assigns to the (already registered) output scatter, s. Preserves the path information of the target.
◆ divide() [5/6]
void Rivet::Analysis::divide |
( |
const YODA::Profile2D & |
p1, |
|
|
const YODA::Profile2D & |
p2, |
|
|
Scatter3DPtr |
s |
|
) |
| const |
Helper for 2D profile histogram division with raw YODA objects
- Note
- Assigns to the (already registered) output scatter, s. Preserves the path information of the target.
◆ divide() [6/6]
void Rivet::Analysis::divide |
( |
CounterPtr |
c1, |
|
|
CounterPtr |
c2, |
|
|
Scatter1DPtr |
s |
|
) |
| const |
Helper for counter division.
- Note
- Assigns to the (already registered) output scatter, s. Preserves the path information of the target.
◆ efficiency() [1/2]
void Rivet::Analysis::efficiency |
( |
const YODA::Histo1D & |
h1, |
|
|
const YODA::Histo1D & |
h2, |
|
|
Scatter2DPtr |
s |
|
) |
| const |
Helper for histogram efficiency calculation.
- Note
- Assigns to the (already registered) output scatter, s. Preserves the path information of the target.
◆ efficiency() [2/2]
void Rivet::Analysis::efficiency |
( |
Histo1DPtr |
h1, |
|
|
Histo1DPtr |
h2, |
|
|
Scatter2DPtr |
s |
|
) |
| const |
Helper for histogram efficiency calculation.
- Note
- Assigns to the (already registered) output scatter, s. Preserves the path information of the target.
◆ integrate() [1/2]
void Rivet::Analysis::integrate |
( |
const Histo1D & |
h, |
|
|
Scatter2DPtr |
s |
|
) |
| const |
Helper for converting a differential histo to an integral one.
- Note
- Assigns to the (already registered) output scatter, s. Preserves the path information of the target.
◆ integrate() [2/2]
void Rivet::Analysis::integrate |
( |
Histo1DPtr |
h, |
|
|
Scatter2DPtr |
s |
|
) |
| const |
Helper for converting a differential histo to an integral one.
- Note
- Assigns to the (already registered) output scatter, s. Preserves the path information of the target.
◆ normalize() [1/2]
template<std::size_t array_size>
void Rivet::Analysis::normalize |
( |
const Histo1DPtr(&) |
histos[array_size], |
|
|
CounterAdapter |
norm = 1.0 , |
|
|
bool |
includeoverflows = true |
|
) |
| |
|
inline |
◆ normalize() [2/2]
void Rivet::Analysis::normalize |
( |
const std::vector< Histo1DPtr > & |
histos, |
|
|
CounterAdapter |
norm = 1.0 , |
|
|
bool |
includeoverflows = true |
|
) |
| |
|
inline |
Normalize the given histograms, histos, to area = norm. - Note
- Constness intentional, if weird, to allow passing rvalue refs of smart ptrs (argh)
- Todo:
- Use SFINAE for a generic iterable of Histo1DPtrs
References Rivet::Analysis::normalize().
◆ scale() [1/2]
template<std::size_t array_size>
void Rivet::Analysis::scale |
( |
const CounterPtr(&) |
cnts[array_size], |
|
|
CounterAdapter |
factor |
|
) |
| |
|
inline |
◆ scale() [2/2]
void Rivet::Analysis::scale |
( |
const std::vector< CounterPtr > & |
cnts, |
|
|
CounterAdapter |
factor |
|
) |
| |
|
inline |
Multiplicatively scale the given counters, cnts, by factor factor. - Note
- Constness intentional, if weird, to allow passing rvalue refs of smart ptrs (argh)
- Todo:
- Use SFINAE for a generic iterable of CounterPtrs
References Rivet::Analysis::scale().
|