|
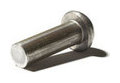 |
Rivet 4.1.0
|
1#ifndef RIVET_MATH_VECTOR2
2#define RIVET_MATH_VECTOR2
4#include "Rivet/Math/MathConstants.hh"
5#include "Rivet/Math/MathUtils.hh"
6#include "Rivet/Math/VectorN.hh"
12 typedef Vector2 TwoVector;
17 Vector2 multiply( const double, const Vector2&);
18 Vector2 multiply( const Vector2&, const double);
19 Vector2 add( const Vector2&, const Vector2&);
20 Vector2 operator*( const double, const Vector2&);
21 Vector2 operator*( const Vector2&, const double);
22 Vector2 operator/( const Vector2&, const double);
23 Vector2 operator+( const Vector2&, const Vector2&);
24 Vector2 operator-( const Vector2&, const Vector2&);
39 template< typename V2TYPE>
41 this->setX(other.x());
42 this->setY(other.y());
46 this->setX(other.get(0));
47 this->setY(other.get(1));
66 double x() const { return get(0); }
67 double y() const { return get(1); }
68 Vector2& setX( double x) { set(0, x); return * this; }
69 Vector2& setY( double y) { set(1, y); return * this; }
74 return _vec.dot(v._vec);
80 if (localDotOther > 1.0) return 0.0;
81 if (localDotOther < -1.0) return M_PI;
82 return acos(localDotOther);
89 if ( md <= 0.0 ) return Vector2();
90 else return * this * 1.0/md;
100 Vector2& operator*=( const double a) {
101 _vec = multiply(a, * this)._vec;
105 Vector2& operator/=( const double a) {
106 _vec = multiply(1.0/a, * this)._vec;
110 Vector2& operator+=( const Vector2& v) {
111 _vec = add(* this, v)._vec;
115 Vector2& operator-=( const Vector2& v) {
116 _vec = subtract(* this, v)._vec;
120 Vector2 operator-() const {
130 inline double dot( const Vector2& a, const Vector2& b) {
134 inline Vector2 multiply( const double a, const Vector2& v) {
136 result._vec = a * v._vec;
140 inline Vector2 multiply( const Vector2& v, const double a) {
141 return multiply(a, v);
144 inline Vector2 operator*( const double a, const Vector2& v) {
145 return multiply(a, v);
148 inline Vector2 operator*( const Vector2& v, const double a) {
149 return multiply(a, v);
152 inline Vector2 operator/( const Vector2& v, const double a) {
153 return multiply(1.0/a, v);
156 inline Vector2 add( const Vector2& a, const Vector2& b) {
158 result._vec = a._vec + b._vec;
162 inline Vector2 subtract( const Vector2& a, const Vector2& b) {
164 result._vec = a._vec - b._vec;
168 inline Vector2 operator+( const Vector2& a, const Vector2& b) {
172 inline Vector2 operator-( const Vector2& a, const Vector2& b) {
173 return subtract(a, b);
Two-dimensional specialisation of Vector. Definition Vector2.hh:28
double dot(const Vector2 &v) const Dot-product with another vector. Definition Vector2.hh:73
Vector2 unit() const Synonym for unitVec. Definition Vector2.hh:94
Vector2 unitVec() const Unit-normalized version of this vector. Definition Vector2.hh:87
double angle(const Vector2 &v) const Angle in radians to another vector. Definition Vector2.hh:78
A minimal base class for -dimensional vectors. Definition VectorN.hh:23
double mod() const Calculate the modulus of a vector. . Definition VectorN.hh:95
Vector< N > & set(const size_t index, const double value) Set indexed value. Definition VectorN.hh:60
Definition MC_CENT_PPB_Projections.hh:10
double angle(const Vector2 &a, const Vector2 &b) Angle (in radians) between two 2-vectors. Definition Vector2.hh:177
|