|
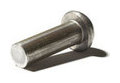 |
Rivet 4.1.0
|
1#ifndef RIVET_PARTICLENAME_HH
2#define RIVET_PARTICLENAME_HH
4#include "Rivet/Particle.fhh"
5#include "Rivet/Tools/Exceptions.hh"
19 constexpr PdgId ANY = 10000;
23 constexpr PdgId ELECTRON = 11;
24 constexpr PdgId POSITRON = -ELECTRON;
25 constexpr PdgId EMINUS = ELECTRON;
26 constexpr PdgId EPLUS = POSITRON;
27 constexpr PdgId MUON = 13;
28 constexpr PdgId ANTIMUON = -MUON;
29 constexpr PdgId TAU = 15;
30 constexpr PdgId ANTITAU = -TAU;
35 constexpr PdgId NU_E = 12;
36 constexpr PdgId NU_EBAR = -NU_E;
37 constexpr PdgId NU_MU = 14;
38 constexpr PdgId NU_MUBAR = -NU_MU;
39 constexpr PdgId NU_TAU = 16;
40 constexpr PdgId NU_TAUBAR = -NU_TAU;
45 constexpr PdgId PHOTON = 22;
46 constexpr PdgId GAMMA = PHOTON;
47 constexpr PdgId GLUON = 21;
48 constexpr PdgId WPLUSBOSON = 24;
49 constexpr PdgId WMINUSBOSON = -WPLUSBOSON;
50 constexpr PdgId WPLUS = WPLUSBOSON;
51 constexpr PdgId WMINUS = WMINUSBOSON;
52 constexpr PdgId WBOSON = WPLUSBOSON;
53 constexpr PdgId Z0BOSON = 23;
54 constexpr PdgId ZBOSON = Z0BOSON;
55 constexpr PdgId Z0 = Z0BOSON;
56 constexpr PdgId HIGGSBOSON = 25;
57 constexpr PdgId HIGGS = HIGGSBOSON;
58 constexpr PdgId H0BOSON = HIGGSBOSON;
59 constexpr PdgId HBOSON = HIGGSBOSON;
64 constexpr PdgId DQUARK = 1;
65 constexpr PdgId UQUARK = 2;
66 constexpr PdgId SQUARK = 3;
67 constexpr PdgId CQUARK = 4;
68 constexpr PdgId BQUARK = 5;
69 constexpr PdgId TQUARK = 6;
74 constexpr PdgId PROTON = 2212;
75 constexpr PdgId ANTIPROTON = -PROTON;
76 constexpr PdgId PBAR = ANTIPROTON;
77 constexpr PdgId NEUTRON = 2112;
78 constexpr PdgId ANTINEUTRON = -NEUTRON;
83 constexpr PdgId PI0 = 111;
84 constexpr PdgId PIPLUS = 211;
85 constexpr PdgId PIMINUS = -PIPLUS;
86 constexpr PdgId RHO0 = 113;
87 constexpr PdgId RHOPLUS = 213;
88 constexpr PdgId RHOMINUS = -RHOPLUS;
89 constexpr PdgId K0L = 130;
90 constexpr PdgId K0S = 310;
91 constexpr PdgId K0 = 311;
92 constexpr PdgId KPLUS = 321;
93 constexpr PdgId KMINUS = -KPLUS;
94 constexpr PdgId ETA = 221;
95 constexpr PdgId ETAPRIME = 331;
96 constexpr PdgId PHI = 333;
97 constexpr PdgId OMEGA = 223;
102 constexpr PdgId ETAC = 441;
103 constexpr PdgId JPSI = 443;
104 constexpr PdgId PSI2S = 100443;
109 constexpr PdgId D0 = 421;
110 constexpr PdgId D0BAR = -421;
111 constexpr PdgId DPLUS = 411;
112 constexpr PdgId DMINUS = -DPLUS;
113 constexpr PdgId DSTARPLUS = 413;
114 constexpr PdgId DSTARMINUS = -DSTARPLUS;
115 constexpr PdgId DSPLUS = 431;
116 constexpr PdgId DSMINUS = -DSPLUS;
121 constexpr PdgId ETAB = 551;
122 constexpr PdgId UPSILON1S = 553;
123 constexpr PdgId UPSILON2S = 100553;
124 constexpr PdgId UPSILON3S = 200553;
125 constexpr PdgId UPSILON4S = 300553;
130 constexpr PdgId B0 = 511;
131 constexpr PdgId B0BAR = -511;
132 constexpr PdgId BPLUS = 521;
133 constexpr PdgId BMINUS = -BPLUS;
134 constexpr PdgId B0S = 531;
135 constexpr PdgId BCPLUS = 541;
136 constexpr PdgId BCMINUS = -BCPLUS;
141 constexpr PdgId LAMBDA = 3122;
142 constexpr PdgId SIGMA0 = 3212;
143 constexpr PdgId SIGMAPLUS = 3222;
144 constexpr PdgId SIGMAMINUS = 3112;
145 constexpr PdgId SIGMAB = 5212;
146 constexpr PdgId SIGMABPLUS = 5222;
147 constexpr PdgId SIGMABMINUS = 5112;
148 constexpr PdgId LAMBDACPLUS = 4122;
149 constexpr PdgId LAMBDACMINUS = -4122;
150 constexpr PdgId LAMBDAB = 5122;
151 constexpr PdgId XI0 = 3322;
152 constexpr PdgId XIMINUS = 3312;
153 constexpr PdgId XIPLUS = -XIMINUS;
154 constexpr PdgId XI0B = 5232;
155 constexpr PdgId XIBMINUS = 5132;
156 constexpr PdgId XI0C = 4132;
157 constexpr PdgId XICPLUS = 4232;
158 constexpr PdgId OMEGAMINUS = 3334;
159 constexpr PdgId OMEGAPLUS = -OMEGAMINUS;
160 constexpr PdgId OMEGABMINUS = 5332;
161 constexpr PdgId OMEGA0C = 4332;
166 constexpr PdgId REGGEON = 110;
167 constexpr PdgId POMERON = 990;
168 constexpr PdgId ODDERON = 9990;
169 constexpr PdgId GRAVITON = 39;
170 constexpr PdgId NEUTRALINO1 = 1000022;
171 constexpr PdgId GRAVITINO = 1000039;
172 constexpr PdgId GLUINO = 1000021;
173 constexpr int BPRIME = 7;
174 constexpr int TPRIME = 8;
175 constexpr int LPRIME = 17;
176 constexpr int NUPRIME = 18;
185 constexpr PdgId DEUTERON = 1000010020;
186 constexpr PdgId ALUMINIUM = 1000130270;
187 constexpr PdgId COPPER = 1000290630;
188 constexpr PdgId XENON = 1000541290;
189 constexpr PdgId GOLD = 1000791970;
190 constexpr PdgId LEAD = 1000822080;
191 constexpr PdgId URANIUM = 1000922380;
200 class ParticleNames {
203 static std::string particleName(PdgId pid) {
204 if (!_instance) _instance = unique_ptr<ParticleNames>( new ParticleNames);
205 return _instance->_particleName( pid);
208 static PdgId particleID( const std::string& pname) {
209 if (!_instance) _instance = unique_ptr<ParticleNames>( new ParticleNames);
210 return _instance->_particleID(pname);
213 std::string _particleName(PdgId pid);
215 PdgId _particleID( const std::string& pname);
222 _add_pid_name(ELECTRON, "ELECTRON");
223 _add_pid_name(POSITRON, "POSITRON");
224 _add_pid_name(MUON, "MUON");
225 _add_pid_name(ANTIMUON, "ANTIMUON");
226 _add_pid_name(TAU, "TAU");
227 _add_pid_name(ANTITAU, "ANTITAU");
229 _add_pid_name(NU_E, "NU_E");
230 _add_pid_name(NU_EBAR, "NU_EBAR");
231 _add_pid_name(NU_MU, "NU_MU");
232 _add_pid_name(NU_MUBAR, "NU_MUBAR");
233 _add_pid_name(NU_TAU, "NU_TAU");
234 _add_pid_name(NU_TAUBAR, "NU_TAUBAR");
236 _add_pid_name(GLUON, "GLUON");
237 _add_pid_name(PHOTON, "PHOTON");
238 _add_pid_name(WPLUSBOSON, "WPLUSBOSON");
239 _add_pid_name(WMINUSBOSON, "WMINUSBOSON");
240 _add_pid_name(ZBOSON, "ZBOSON");
241 _add_pid_name(HIGGS, "HIGGS");
243 _add_pid_name(DQUARK, "DOWN");
244 _add_pid_name(UQUARK, "UP");
245 _add_pid_name(SQUARK, "STRANGE");
246 _add_pid_name(CQUARK, "CHARM");
247 _add_pid_name(BQUARK, "BOTTOM");
248 _add_pid_name(TQUARK, "TOP");
249 _add_pid_name(-DQUARK, "ANTIDOWN");
250 _add_pid_name(-UQUARK, "ANTIUP");
251 _add_pid_name(-SQUARK, "ANTISTRANGE");
252 _add_pid_name(-CQUARK, "ANTICHARM");
253 _add_pid_name(-BQUARK, "ANTIBOTTOM");
254 _add_pid_name(-TQUARK, "ANTITOP");
256 _add_pid_name(PROTON, "PROTON");
257 _add_pid_name(ANTIPROTON, "ANTIPROTON");
258 _add_pid_name(NEUTRON, "NEUTRON");
259 _add_pid_name(ANTINEUTRON, "ANTINEUTRON");
261 _add_pid_name(PI0, "PI0");
262 _add_pid_name(PIPLUS, "PIPLUS");
263 _add_pid_name(PIMINUS, "PIMINUS");
264 _add_pid_name(RHO0, "RHO0");
265 _add_pid_name(RHOPLUS, "RHOPLUS");
266 _add_pid_name(RHOMINUS, "RHOMINUS");
267 _add_pid_name(K0, "K0");
268 _add_pid_name(KPLUS, "KPLUS");
269 _add_pid_name(KMINUS, "KMINUS");
270 _add_pid_name(ETA, "ETA");
271 _add_pid_name(PHI, "PHI");
273 _add_pid_name(D0, "D0");
274 _add_pid_name(D0BAR, "D0BAR");
275 _add_pid_name(DPLUS, "DPLUS");
276 _add_pid_name(DMINUS, "DMINUS");
278 _add_pid_name(B0, "B0");
279 _add_pid_name(B0BAR, "B0BAR");
280 _add_pid_name(BPLUS, "BPLUS");
281 _add_pid_name(BMINUS, "BMINUS");
283 _add_pid_name(LAMBDA, "LAMBDA");
284 _add_pid_name(SIGMA0, "SIGMA0");
285 _add_pid_name(SIGMAPLUS, "SIGMAPLUS");
286 _add_pid_name(SIGMAMINUS, "SIGMAMINUS");
287 _add_pid_name(XI0, "XI0");
288 _add_pid_name(XIPLUS, "XIPLUS");
289 _add_pid_name(XIMINUS, "XIMINUS");
291 _add_pid_name(REGGEON, "REGGEON");
292 _add_pid_name(POMERON, "POMERON");
294 _add_pid_name(DEUTERON, "DEUTERON");
295 _add_pid_name(ALUMINIUM, "ALUMINIUM");
296 _add_pid_name(COPPER, "COPPER");
297 _add_pid_name(XENON, "XENON");
298 _add_pid_name(GOLD, "GOLD");
299 _add_pid_name(LEAD, "LEAD");
300 _add_pid_name(URANIUM, "URANIUM");
302 _add_pid_name(ANY, "*");
305 void _add_pid_name(PdgId pid, const std::string& pname) {
306 _ids_names[ pid] = pname;
307 _names_ids[pname] = pid;
310 static unique_ptr<ParticleNames> _instance;
312 std::map<PdgId, std::string> _ids_names;
314 std::map<std::string, PdgId> _names_ids;
320 inline std::string toParticleName(PdgId p) {
321 return ParticleNames::particleName(p);
326 inline PdgId toParticleID( const std::string& pname) {
327 return ParticleNames::particleID(pname);
332 inline std::pair<PdgId,PdgId> make_pdgid_pair(PdgId a, PdgId b) {
333 return make_pair(a, b);
338 inline std::pair<PdgId,PdgId> make_pdgid_pair( const std::string& a, const std::string& b) {
339 const PdgId pa = toParticleID(a);
340 const PdgId pb = toParticleID(b);
341 return make_pair(pa, pb);
346 inline std::string toBeamsString( const PdgIdPair& pair) {
348 toParticleName(pair.first) + ", " +
349 toParticleName(pair.second) + "]";
int pid(const Particle &p) Unbound function access to PID code. Definition ParticleUtils.hh:23
Definition MC_CENT_PPB_Projections.hh:10
|