|
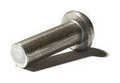 |
Rivet 4.0.0
|
1#ifndef RIVET_MATH_MATRIXN
2#define RIVET_MATH_MATRIXN
4#include "Rivet/Math/MathConstants.hh"
5#include "Rivet/Math/MathUtils.hh"
6#include "Rivet/Math/Vectors.hh"
8#include "Rivet/Math/eigen3/Dense"
15 typedef Matrix<4> Matrix4;
18 Matrix<N> multiply( const Matrix<N>& a, const Matrix<N>& b);
20 Matrix<N> divide( const Matrix<N>&, const double);
22 Matrix<N> operator*( const Matrix<N>& a, const Matrix<N>& b);
53 for ( size_t i = 0; i < N; ++i) {
54 rtn.set(i, i, diag[i]);
61 for ( size_t i = 0; i < N; ++i) {
70 Matrix() : _matrix(EMatrix::Zero()) {}
72 Matrix& set( const size_t i, const size_t j, const double value) {
74 _matrix(i, j) = value;
76 throw std::runtime_error( "Attempted set access outside matrix bounds.");
81 double get( const size_t i, const size_t j) const {
85 throw std::runtime_error( "Attempted get access outside matrix bounds.");
89 Vector<N> getRow( const size_t row) const {
91 for ( size_t i = 0; i < N; ++i) {
92 rtn. set(i, _matrix(row,i));
98 for ( size_t i = 0; i < N; ++i) {
99 _matrix(row,i) = r.get(i);
104 Vector<N> getColumn( const size_t col) const {
106 for ( size_t i = 0; i < N; ++i) {
107 rtn. set(i, _matrix(i,col));
113 for ( size_t i = 0; i < N; ++i) {
114 _matrix(i,col) = c.get(i);
121 tmp._matrix = _matrix.transpose();
133 tmp._matrix = _matrix. inverse();
139 return _matrix.determinant();
145 for ( size_t i = 0; i < N; ++i) {
155 rtn._matrix = -_matrix;
160 constexpr size_t size() const {
165 bool isZero( double tolerance=1E-5) const {
166 for ( size_t i=0; i < N; ++i) {
167 for ( size_t j=0; j < N; ++j) {
176 for ( size_t i=0; i < N; ++i) {
177 for ( size_t j=i; j < N; ++j) {
178 if (! Rivet::isZero(_matrix(i,j) - other._matrix(i,j)) ) return false;
186 return isEqual(this->transpose());
191 for ( size_t i=0; i < N; ++i) {
192 for ( size_t j=0; j < N; ++j) {
193 if (i == j) continue;
200 bool operator == ( const Matrix<N>& a) const {
201 return _matrix == a._matrix;
204 bool operator != ( const Matrix<N>& a) const {
205 return _matrix != a._matrix;
224 Matrix<N>& operator *= ( const Matrix<N>& m) {
225 _matrix *= m._matrix;
229 Matrix<N>& operator *= ( const double a) {
234 Matrix<N>& operator /= ( const double a) {
239 Matrix<N>& operator += ( const Matrix<N>& m) {
240 _matrix += m._matrix;
244 Matrix<N>& operator -= ( const Matrix<N>& m) {
245 _matrix -= m._matrix;
251 using EMatrix = RivetEigen::Matrix<double,N,N>;
261 inline Matrix<N> add( const Matrix<N>& a, const Matrix<N>& b) {
263 result._matrix = a._matrix + b._matrix;
268 inline Matrix<N> subtract( const Matrix<N>& a, const Matrix<N>& b) {
273 inline Matrix<N> operator + ( const Matrix<N> a, const Matrix<N>& b) {
278 inline Matrix<N> operator - ( const Matrix<N> a, const Matrix<N>& b) {
279 return subtract(a, b);
283 inline Matrix<N> multiply( const double a, const Matrix<N>& m) {
285 rtn._matrix = a * m._matrix;
290 inline Matrix<N> multiply( const Matrix<N>& m, const double a) {
291 return multiply(a, m);
295 inline Matrix<N> divide( const Matrix<N>& m, const double a) {
296 return multiply(1/a, m);
300 inline Matrix<N> operator * ( const double a, const Matrix<N>& m) {
301 return multiply(a, m);
305 inline Matrix<N> operator * ( const Matrix<N>& m, const double a) {
306 return multiply(a, m);
310 inline Matrix<N> multiply( const Matrix<N>& a, const Matrix<N>& b) {
312 tmp._matrix = a._matrix * b._matrix;
317 inline Matrix<N> operator * ( const Matrix<N>& a, const Matrix<N>& b) {
318 return multiply(a, b);
323 inline Vector<N> multiply( const Matrix<N>& a, const Vector<N>& b) {
325 tmp._vec = a._matrix * b._vec;
330 inline Vector<N> operator * ( const Matrix<N>& a, const Vector<N>& b) {
331 return multiply(a, b);
335 inline Matrix<N> transpose( const Matrix<N>& m) {
343 return m.transpose();
347 inline Matrix<N> inverse( const Matrix<N>& m) {
352 inline double det( const Matrix<N>& m) {
353 return m.determinant();
357 inline double trace( const Matrix<N>& m) {
368 std::ostringstream ss;
370 for ( size_t i = 0; i < m.size(); ++i) {
372 for ( size_t j = 0; j < m.size(); ++j) {
373 const double e = m.get(i, j);
397 for ( size_t i = 0; i < N; ++i) {
398 for ( size_t j = 0; j < N; ++j) {
399 const double a = ma.get(i, j);
400 const double b = mb.get(i, j);
411 return m.isZero(tolerance);
General -dimensional mathematical matrix object. Definition MatrixN.hh:30
double trace() const Calculate trace. Definition MatrixN.hh:143
double det() const Calculate determinant. Definition MatrixN.hh:138
Matrix< N > operator-() const Negate. Definition MatrixN.hh:153
bool isZero(double tolerance=1E-5) const Index-wise check for nullness, allowing for numerical precision. Definition MatrixN.hh:165
constexpr size_t size() const Get dimensionality. Definition MatrixN.hh:160
bool isDiag() const Check that all off-diagonal elements are zero, allowing for numerical precision. Definition MatrixN.hh:190
Matrix< N > inverse() const Calculate inverse. Definition MatrixN.hh:131
bool isSymm() const Check for symmetry under transposition. Definition MatrixN.hh:185
bool isEqual(Matrix< N > other) const Check for index-wise equality, allowing for numerical precision. Definition MatrixN.hh:175
A minimal base class for -dimensional vectors. Definition VectorN.hh:23
Vector< N > & set(const size_t index, const double value) Set indexed value. Definition VectorN.hh:60
Definition MC_CENT_PPB_Projections.hh:10
std::ostream & operator<<(std::ostream &os, const AnalysisInfo &ai) Stream an AnalysisInfo as a text description. Definition AnalysisInfo.hh:362
std::enable_if< std::is_arithmetic< N1 >::value &&std::is_arithmetic< N2 >::value &&(std::is_floating_point< N1 >::value||std::is_floating_point< N2 >::value), bool >::type fuzzyEquals(N1 a, N2 b, double tolerance=1e-5) Compare two numbers for equality with a degree of fuzziness. Definition MathUtils.hh:57
std::string toString(const AnalysisInfo &ai) String representation.
std::enable_if< std::is_floating_point< NUM >::value, bool >::type isZero(NUM val, double tolerance=1e-8) Compare a number to zero. Definition MathUtils.hh:24
|