|
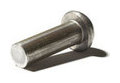 |
Rivet 4.0.0
|
2#ifndef RIVET_DileptonFinder_HH
3#define RIVET_DileptonFinder_HH
5#include "Rivet/Projections/FinalState.hh"
6#include "Rivet/Projections/LeptonFinder.hh"
7#include "Rivet/Projections/VetoedFinalState.hh"
24 const Cut& lcuts=Cuts::OPEN,
25 const Cut& llcuts=Cuts::OPEN,
28 TauDecaysAs tauDecays=TauDecaysAs::PROMPT,
29 MuDecaysAs muDecays=MuDecaysAs::PROMPT,
37 const Cut& lcuts=Cuts::OPEN,
38 const Cut& llcuts=Cuts::OPEN,
47 const Cut& lcuts=Cuts::OPEN,
48 const Cut& llcuts=Cuts::OPEN,
59 double minmass, double maxmass,
63 Cuts:: mass >= minmass && Cuts:: mass < maxmass)
74 const Cut& lcuts=Cuts::OPEN,
75 double minmass=-DBL_MAX, double maxmass=DBL_MAX)
78 Cuts:: mass >= minmass && Cuts:: mass < maxmass)
88 const Cut& lcuts=Cuts::OPEN,
89 double minmass=-DBL_MAX, double maxmass=DBL_MAX,
92 TauDecaysAs tauDecays=TauDecaysAs::PROMPT,
93 MuDecaysAs muDecays=MuDecaysAs::PROMPT,
97 Cuts:: mass >= minmass && Cuts:: mass < maxmass,
98 whichleptons, whichphotons, tauDecays, muDecays, dressing)
107 const Cut& lcuts=Cuts::OPEN,
108 double minmass=-DBL_MAX, double maxmass=DBL_MAX,
111 TauDecaysAs tauDecays=TauDecaysAs::PROMPT,
112 MuDecaysAs muDecays=MuDecaysAs::PROMPT,
115 Cuts:: mass >= minmass && Cuts:: mass < maxmass,
116 whichleptons, whichphotons, tauDecays, muDecays, dressing)
126 const Cut& lcuts=Cuts::OPEN,
127 double minmass=-DBL_MAX, double maxmass=DBL_MAX)
129 Cuts:: mass >= minmass && Cuts:: mass < maxmass)
179 void clear() { _theParticles.clear(); }
182 using Projection::operator =;
Convenience finder of leptonically decaying Zs. Definition DileptonFinder.hh:15
const Particles & bosons() const Access to the found bosons. Definition DileptonFinder.hh:142
const VetoedFinalState & remainingFinalState() const
void project(const Event &e) Apply the projection on the supplied event.
DileptonFinder(const FinalState &allfs, const Cut &lcuts, double masstarget, PdgId pid, double minmass, double maxmass, double dRdress) Old constructor taking min and max ll masses. Definition DileptonFinder.hh:55
Particles leptons() const Access to the constituent clustered leptons (and photons) Definition DileptonFinder.hh:158
DileptonFinder(const FinalState &allfs, PdgId pid, double masstarget, double dRdress, const Cut &lcuts=Cuts::OPEN, double minmass=-DBL_MAX, double maxmass=DBL_MAX) Definition DileptonFinder.hh:70
const Particle & boson() const Access to the found boson (assuming it exists). Definition DileptonFinder.hh:144
DileptonFinder(const FinalState &allfs, double masstarget, double dRdress, const Cut &lcuts=Cuts::OPEN, const Cut &llcuts=Cuts::OPEN, DressingType dressing=DressingType::CONE) Modern constructor with an explicit FS, explicit mass target and dressing dR up-front (PID and LL via...
RIVET_DEFAULT_PROJ_CLONE(DileptonFinder) Clone on the heap.
DileptonFinder(double masstarget, double dRdress, const Cut &lcuts=Cuts::OPEN, double minmass=-DBL_MAX, double maxmass=DBL_MAX, LeptonOrigin whichleptons=LeptonOrigin::PROMPT, PhotonOrigin whichphotons=PhotonOrigin::NODECAY, TauDecaysAs tauDecays=TauDecaysAs::PROMPT, MuDecaysAs muDecays=MuDecaysAs::PROMPT, DressingType dressing=DressingType::CONE) Definition DileptonFinder.hh:105
DileptonFinder(PdgId pid, double masstarget, double dRdress, const Cut &lcuts=Cuts::OPEN, double minmass=-DBL_MAX, double maxmass=DBL_MAX, LeptonOrigin whichleptons=LeptonOrigin::PROMPT, PhotonOrigin whichphotons=PhotonOrigin::NODECAY, TauDecaysAs tauDecays=TauDecaysAs::PROMPT, MuDecaysAs muDecays=MuDecaysAs::PROMPT, DressingType dressing=DressingType::CONE) Definition DileptonFinder.hh:85
const Particles & constituents() const Access to the constituent clustered leptons (and photons)
CmpState compare(const Projection &p) const Compare projections.
DileptonFinder(const FinalState &allfs, double masstarget, double dRdress, const Cut &lcuts=Cuts::OPEN, double minmass=-DBL_MAX, double maxmass=DBL_MAX) Definition DileptonFinder.hh:123
DileptonFinder(double masstarget, double dRdress, const Cut &lcuts=Cuts::OPEN, const Cut &llcuts=Cuts::OPEN, LeptonOrigin leptonOrigin=LeptonOrigin::PROMPT, PhotonOrigin photonOrigin=PhotonOrigin::NODECAY, TauDecaysAs tauDecays=TauDecaysAs::PROMPT, MuDecaysAs muDecays=MuDecaysAs::PROMPT, DressingType dressing=DressingType::CONE) Modern constructor with a default FS, explicit mass target and dressing dR up-front (PID and LL via c...
DileptonFinder(const FinalState &leptonfs, const FinalState &photonfs, double masstarget, double dRmax, const Cut &lcuts=Cuts::OPEN, const Cut &llcuts=Cuts::OPEN, DressingType dressing=DressingType::CONE) Modern constructor with two explicit FSes, explicit mass target and dressing dR up-front (PID and LL ...
void clear() Clear the projection. Definition DileptonFinder.hh:179
Representation of a HepMC event, and enabler of Projection caching. Definition Event.hh:22
Project out all final-state particles in an event. Probably the most important projection in Rivet! Definition FinalState.hh:12
virtual const Particles & particles() const Get the particles in no particular order, with no cuts. Definition ParticleFinder.hh:65
Particle representation, either from a HepMC::GenEvent or reconstructed. Definition Particle.hh:45
Specialised vector of Particle objects. Definition Particle.hh:21
Base class for all Rivet projections. Definition Projection.hh:29
FS modifier to exclude classes of particles from the final state. Definition VetoedFinalState.hh:11
double mass(const FourMomentum &a, const FourMomentum &b) Calculate mass of two 4-vectors. Definition Vector4.hh:1461
int pid(const Particle &p) Unbound function access to PID code. Definition ParticleUtils.hh:23
int abspid(const Particle &p) Unbound function access to abs PID code. Definition ParticleUtils.hh:26
Definition MC_CENT_PPB_Projections.hh:10
PhotonOrigin Accepted classes of lepton origin. Definition DressedLepton.hh:24
DressingType The approach taken to photon dressing of leptons. Definition DressedLepton.hh:21
LeptonOrigin Accepted classes of lepton origin. Definition DressedLepton.hh:12
|